
CBSE Guess > Papers > Important Questions > Class XI > 2012 > Informatics Practices By Ms. Lakshmi Pulikollu
CBSE CLASS XI
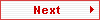
Chapter 5
(a) Design a GUI desktop application in java to accept the side of a square in a text field and calculate the area and perimeter of the square. Display the results in two separate text fields. Add appropriate labels and an exit button to end the application.
Code :
private void CalculateActionPerformed(java.awt.event.ActionEvent evt) {
double s=Double.parseDouble(tf1.getText());
double a= s*s;
double p =4 *s;
tf2.setText(""+a);
tf3.setText(""+p); // TODO add your handling code here:
}
private void ExitActionPerformed(java.awt.event.ActionEvent evt) {
System.exit(0); // TODO add your handling code here:
}
Output:
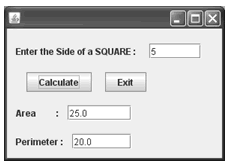
(b) Design a GUI desktop application in java to accept marks in 5 subjects in five text fields and calculate the total and average marks. Display the results in separate text fields, which are disabled. Add appropriate labels and an exit button to end the application.
Code :
private void CalculateActionPerformed(java.awt.event.ActionEvent evt) {
double m1=Double.parseDouble(tf1.getText());
double m2=Double.parseDouble(tf2.getText());
double m3=Double.parseDouble(tf3.getText());
double m4=Double.parseDouble(tf4.getText());
double m5=Double.parseDouble(tf5.getText());
double tot= m1+m2+m3+m4+m5;
double avg= tot/5;
tf6.setEnabled(false);
tf7.setEnabled(false);
tf6.setText(""+tot);
tf7.setText(""+avg); // TODO add your handling code here:
}
private void ExitActionPerformed(java.awt.event.ActionEvent evt) {
System.exit(0); // TODO add your handling code here:
}
Output :
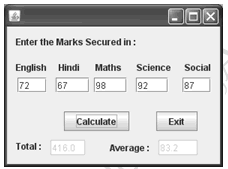
(c) Design a GUI desktop application in java to accept sales of a company for four quarters in text fields. Calculate the total yearly sale and display the same in a dialog box. Add appropriate labels and an exit button to end the application.
Code :
import javax.swing.JOptionPane;
private void CalculateActionPerformed(java.awt.event.ActionEvent evt) {
double s1=Double.parseDouble(tf1.getText());
double s2=Double.parseDouble(tf2.getText());
double s3=Double.parseDouble(tf3.getText());
double s4=Double.parseDouble(tf4.getText());
double tot= s1+s2+s3+s4;
JOptionPane.showMessageDialog(null,"Yearly Sales = "+tot);
}
private void ExitActionPerformed(java.awt.event.ActionEvent evt) {
System.exit(0); // TODO add your handling code here:
}
Output :
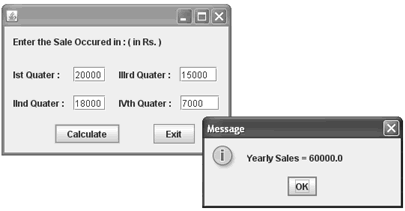
(d) Design a GUI desktop application in java to accept length in kilometers in a text field and display the converted length in meters in a second text field which is disabled. Add appropriate labels and an exit button to end the application
Code :
private void ConvertActionPerformed(java.awt.event.ActionEvent evt) {
double len=Double.parseDouble(tf1.getText());
double len1= len*1000; //1Km = 1000 mtrs
tf2.setEnabled(false);
tf2.setText(""+len1); // TODO add your handling code here:
}
private void ExitActionPerformed(java.awt.event.ActionEvent evt) {
System.exit(0); // TODO add your handling code here:
}
Output :
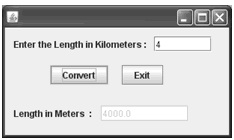
(e) Design a GUI desktop application in java to accept two numbers in a in a text field and Interchange the values of first number and second number using a temporary variable. Add appropriate labels and an exit button to end the application.
Code :
private void SwapActionPerformed(java.awt.event.ActionEvent evt) {
int fn=Integer.parseInt(tf1.getText());
int sn=Integer.parseInt(tf2.getText());
int temp=fn;
fn=sn;
sn=temp;
tf3.setText(""+fn);
tf4.setText(""+sn); // TODO add your handling code here:
}
private void ExitActionPerformed(java.awt.event.ActionEvent evt) {
System.exit(0); // TODO add your handling code here:
}
Output :
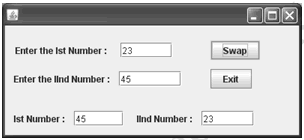
(f) Write the code for the following application
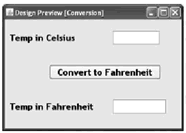
[Hint :Tc = (5/9)*(Tf-32) and Tf = (9/5)*Tc+32 where Tc = temperature in degrees Celsius, Tf = temperature in degrees Fahrenheit]
Code :
private void ConvertActionPerformed(java.awt.event.ActionEvent evt) {
int c=Integer.parseInt(tf1.getText());
int f=(c*9/5)+32;
tf2.setText(""+f); // TODO add your handling code here:
}
(g) Write the code for the following application :
[Hint : Area of Rectangle=Length*Breadth and Perimeter of Rectangle = 2 * ( Length + Breadth ) ]
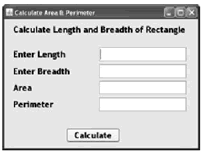
Code :
private void ConvertActionPerformed(java.awt.event.ActionEvent evt) {
int l=Integer.parseInt(tf1.getText());
int b=Integer.parseInt(tf2.getText());
tf3.setText(""+(l*b)); // Area
tf4.setText(""+(2*(l+b))); // Perimeter
}
(h) Write the code for the following application :
[Hint : SI [Interest] = (P×R×T)/100 and Amount = Principle + SI]
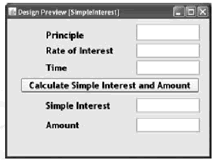
Code :
private void ConvertActionPerformed(java.awt.event.ActionEvent evt) {
double p=Double.parseDouble(tf1.getText());
double r=Double.parseDouble(tf2.getText());
double t=Double.parseDouble(tf3.getText());
double si=(p*t*r)/100;
double amt = p+si;
tf4.setText(""+si);
tf5.setText(""+amt);
}
Submitted By Ms. Lakshmi Pulikollu
Email Id : [email protected] |