
CBSE Guess > Papers > Important Questions > Class XII > 2011 > Computer Science > Computer Science By Deepa
CBSE CLASS XII
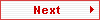
Arrays (Questions with Answer)
Page 3 of 3
- Write a function in C++ to replace the repeating elements in an array by 0 . The zeros should be shifted to the end. The order of the array should not change.
Eg : Array : 10 , 20 , 30 , 10 , 40 , 20 , 30
Result : 10 , 20 , 30 , 40 , 0 , 0 , 0
Ans.
void twodigit(int a[10],int n)
{ int i,j,temp;
for(i=0;i<n;i++)
for(j=i+1;j<n;j++)
{ if(a[i]==a[j])
a[j]=0;
}
for(i=0;i<n-1;i++)
if(a[i]==0)
{
temp=a[i];
a[i]=a[i+1];
a[i+1]=temp;
}
cout<<"\nResult :";
for(i=0;i<n;i++)
cout<<a[i]<<" ";
}
- Write a function in C++ called shift( ) to rearrange the matrix as shown .

Ans.
void shift(int a[10][10],int m,int n)
{
int b[10][10]={0},i,j,k=0,c[10][10]={0};
for(i=0;i<m;i++)
{
k=0;
for(j=0;j<n;j++)
{
if(a[i][j]<0)
{
b[i][k]=a[i][j];
k++;
}
}
} for(i=0;i<m;i++)
{ k=n-1;
for(j=n-1;j>=0;j--)
{
if(a[i][j]>=0)
{ c[i][k]=a[i][j];
k--;
}
}
}
for(i=0;i<m;i++)
for(j=0;j<n;j++)
a[i][j]=b[i][j]+c[i][j];
for(i=0;i<m;i++)
{ cout<<endl;
for(j=0;j<n;j++)
cout<<a[i][j]<<" ";
}
}
- Define a function SWAPARR( ) in c++ to swap the first row elements with the last row elements, for a 2d integer array passed as the argument of the function.

Ans.
void SWAPARR(int a[10][10],int m,int n)
{
int i,j,temp;
for(i=0;i<n;i++)
{ temp=a[0][i];
a[0][i]=a[m-1][i];
a[m-1][i]=temp;}
cout<<"\nThe array after swapping";
for(i=0;i<m;i++)
{ cout<<endl;
for(j=0;j<n;j++)
cout<<a[i][j]<<" ";
}
}
- Write a function in C++ which accepts an integer array M x N and its size as arguments and then displays the array after exchanging the elements in the upper half of the diagonal with the lower half of the diagonal, do not use parallel arrays.
Ans.
void exchange(int a[10][10],int m,int n)
{ int i,j,temp;
for(i=0;i<m;i++)
for(j=0;j<n;j++)
{ if(i>j)
{ temp=a[i][j];
a[i][j]=a[j][i];
a[j][i]=temp;
} }
cout<<"\nThe array after swapping";
for(i=0;i<m;i++)
{ cout<<endl;
for(j=0;j<n;j++)
cout<<a[i][j]<<" ";
}}
- Write a function in C++ which accepts an integer array and its size as arguments and replaces elements having even values with its half and elements having off values with twice its value.
Eg. If an array of five elements initially contains the elements as
3,4, 5, 16, 9
Then the function should rearrange the content of the array as
6, 2, 10, 8, 18
Ans.
void change(int a[10],int n)
{
int i;
for(i=0;i<n;i++)
{
if(a[i]%2==1)
a[i]=a[i]*2;
else if(a[i]%2==0)
a[i]=a[i]/2;
}
for(i=0;i<n;i++)
cout<<a[i]<<" ";
}
- Define a function COPY( ) to copy the last five elements of array B after first 5 elements of array A and store it in the third array C.
Ans.
void extra(int a[10],int b[10],int n)
{
int c[30],counta=0,countb=n-1,countc=0,i;
while(countc<5)
{ c[countc]=a[counta];
counta++;
countc++;
}
while(countc<10)
{ c[countc]=b[countb];
countb--;
countc++;
}
for(i=0;i<10;i++)
cout<<c[i]<<" ";
}
- Write a function which finds the locations and values of largest and second largest element in a two dimensional array with M rows and N columns.
Ans.
void largest(int a[10][10],int m,int n)
{
int i,j,pr1=0,pc1=0,pr2=0,pc2=0,fl=a[0][0],sl=0;
for(i=0;i<m;i++)
for(j=0;j<n;j++)
{
if(a[i][j]>fl)
{
fl=a[i][j];
pr1=i;
pc1=j;
}
if((a[i][j]>sl) && a[i][j]<fl)
{
sl=a[i][j];
pr2=i;
pc2=j;
}
}
cout<<"\nLargest Element is "<<fl<<" and is stored at row "<<pr1<<" and column "<<pc1;
cout<<"\nSecond Largest Element is "<<sl<<" and is stored at row "<<pr2<<" and column "<<pc2;
}
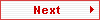
Submitted By Deepa
Email Id : [email protected]
About Author: Computer Teacher From Sunrise English Pvt. School |
|