
CBSE Guess > Papers > Important Questions > Class XII > 2010 > Computer Science > Computer Science By Ravi Kiran
CBSE CLASS XII
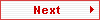
3. (a) Write a function in C++ which accepts an integer array and its size as arguments/parameters and exchanges the values of first half side elements with the second half side elements of the array. 3
Example:
If an array of eight elements has initial content as
2,4,1,6,7,9,23,10
The function should rearrange the array as
7,9,23,10,2,4,1,6
(b) An array Arr[15][35] is stored in the memory along the column with each of its elements occupying 8 bytes. Find out the base address and the address of an element Arr[2][5], if the location Arr[5][10] is stored at the address 4000. 4
(c) Write a function in C++ to perform a PUSH operation in a dynamically allocated
stack considering the following: 4
struct Node
{
int X, Y;
Node *Link;
};
class STACK
{
Node *Top;
public:
STACK() {Top=NULL;}
void PUSH();
void POP() ;
~STACK();
};
(d) Write a function in C++ to print the sum of all the values which are either divisible
by 2 or are divisible by 3 present in a two-dimensional array passed as the
argument to the function. 3
(e) Evaluate the following postfix notation of expression: 2
10 20 + 25 15 - * 30 /
4. (a) Observe the program segment given below carefully, and answer the question that
follows: 1
class Book
{
int Book no;
char Book_name[20];
public:
//function to enter Book details
void enterdetails();
// function to display Book details
void showdetails();
//function to return Book_no
int Rbook_no(){return Book_no;}
} ;
void Modify(Book NEW)
{
fstream File;
File.open(“BOOK.DAT”,ios::binary|ios::in|ios::out);
Book OB;
int Recordsread = 0, Found = 0;
while (!Found && File.read((char*)&OB, sizeof(OB)))
{
Recordsread ++ ;
if (NEW.RBook_no() == OB.RBook_no))
{
______________ //Missing Statement
File.write((char*)&NEW, sizeof (NEW));
Found = 1;
}
else
File.write((char*)&OB, sizeof(OB));
}
if (!Found)
cout<<" Record for modification does not exist”;
File.close();
}
If the function Modify( ) is supposed to modify a record in file BOOK.DAT with the
values of Book NEW passed to its argument, write the appropriate statement for Missing Statement using seekp( ) or seekg( ), whichever needed, in the above code
that would write the modified record at its proper place.
(b) Write a function in C++ to count and display the number of lines starting with
alphabet ‘A’ present in a text file “LINES.TXT”.
Example:
If the file “LINES.TXT” contains the following lines,
A boy is playing there.
There is a playground.
An aeroplane is in the sky.
Alphabets and numbers are allowed in the password.
The function should display the output as 3
(c) Given a binary file STUDENT.DAT, containing records of the following class
Student type 3
class Student
{
char S_Admno[lO]; //Admission number of student
char S_Name[30]; //Name of student
int Percentage; //Marks Percentage of student
public:
void EnterData()
{
gets(S_Admno);gets(S_Name);cin>>Percentage;
}
void DisplayData()
{
cout<<setw(12)<<S_Admno;
cout<<setw(32)<<S_Name;
cout<<setw(3)<<Percentage<<endl;
}
int ReturnPercentage(){return Percentage;}
};
Write a function in C++, that would read contents of file STUDENT.DAT and display
the details of those Students whose Percentage is above
Paper By Mr. Ravi Kiran
Email Id : [email protected] |